- Published on
Cross Origin Resource Sharing (CORS), What is it?
- Authors
- Name
- Fadjar Irfan Rafi
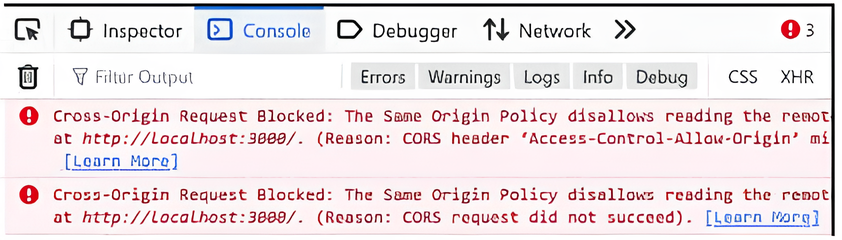
Have you ever encountered this problem while designing a web application? Yes, this is a nightmare for some devs, including myself when I first learned. I discovered this problem when learning how to develop an API for a blog. To understand how this error could happen, we need to know what CORS and how it works.
The Same-Origin Policy
By default, the browser can only make requests if the resource is from the same source (same domain, protocol, or port); this is known as the same-origin policy (SOP). This SOP will limit how scripts in our web application from one origin interact with resources from another.
Imagine you have a web application with the domain 'https://www.domain-a.com' that requires data from an API located at 'https://api.domain-b.com'. Cross-origin requests are those that come from different origins.
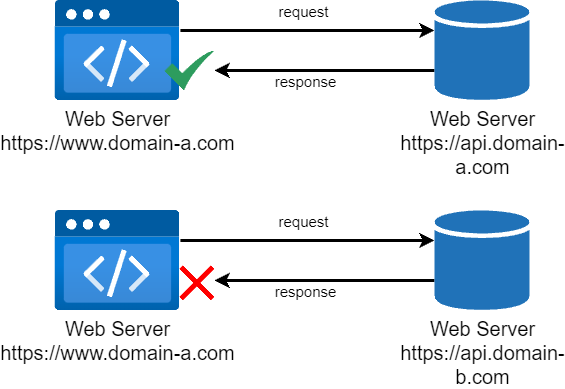
However, what happens if our web application must communicate with sources that are not on our domain? Here's where CORS is put into practice.
What is CORS
Cross-origin resource sharing is a way for browsers manage access to resources from other domains. In order for the browser and the server to communicate, CORS adds HTTP headers to our requests. Which origin is permitted access to the resource is indicated by this HTTP header.
There are several CORS headers, but the main standard headers used are:
Access-Control-Allow-Origin
Access-Control-Allow-Methods
Access-Control-Allow-Headers
When we make a request such as GET
, POST
or HEAD
, the request will be sent from the browser to the server without custom headers.
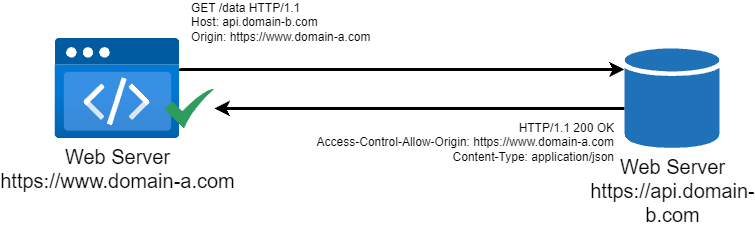
Request from Browser:
GET /data HTTP/1.1
Host: api.domain-b.com
Origin: https://www.domain-a.com
Response from Browser:
HTTP/1.1 200 OK
Access-Control-Allow-Origin: https://www.domain-a.com
Content-Type: application/json
{ "message": "Hello from domain-b!" }
However, if we want to make requests like 'DELETE', 'PUT', 'PATCH', or requests with custom headers, the browser will first do a preflight check to ensure that the original request is safe to send by making a 'OPTION' request. For instance, our program asks to delete data.
OPTIONS
Request from Browser:
Preflight OPTIONS /data HTTP/1.1
Host: api.domain-b.com
Origin: https://www.domain-a.com
Access-Control-Request-Method: DELETE
Access-Control-Request-Headers: Content-Type
OPTIONS
Response from Server:
Preflight HTTP/1.1 204 No Content
Access-Control-Allow-Origin: https://www.domain-a.com
Access-Control-Allow-Methods: GET, POST, DELETE, OPTIONS
Access-Control-Allow-Headers: Content-Type
After successfully receiving a response from preflight, our browser sends the request, and if successful, the server returns an ok response.
DELETE
from Browser:
Request DELETE /data/123 HTTP/1.1
Host: api.domain-b.com
Origin: https://www.domain-a.com
Content-Type: application/json
Response from Browser:
HTTP/1.1 200 OK
Access-Control-Allow-Origin: https://www.domain-a.com
Content-Type: application/json
{"message": "Resource deleted successfully!"}
Why CORS matters?
CORS enables web servers to determine who can access resources. This is important to prevent potential inter-domain attacks, such as cross-site request forgery (CSRF) attacks caused by unauthorized queries to other domains. In addition, CORS allows web applications to safely interact with a wide range of resources from many origins.
Thanks for Reading✌️